CSE 1710.03A Programming for Digital Media
Lab 11
Due date: Nov 30, 2009 at 20:00
Making a Simple Movie
Your job here is to generate the images for a movie by writing a program.
The content for the movie is a simple animation of a smile. The following
two images show the first and last frame of the movie, without
eyes:
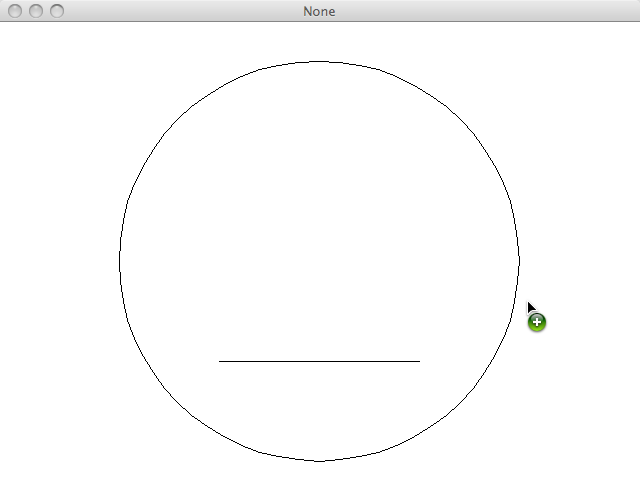
The size of the frames for the movie should be 640x480 and should contain
exactly 80 frames. The function to create the movie should be called
makeSmile().
On each empty frame (which should be created with makeEmptyPicture),
draw a circle with the addOval() function, see the
documentation in help of JES (section 7, "Picture Functions").
The diameter of the circle should be 400 pixels, centered
at the middle of the image (i.e. 320x240).
The mouth should be drawn as two straight lines with the
addLine() function.
The left part of the mouth extends horizontally from pixel position
x=220 to 320, the right part from x=320 to 420.
Initially,
the two lines should be horizontal, for a "neutral" mouth position,
initially at vertical pixel position y=340. At the end of the movie,
i.e. after 80 frames, the left and right corners of the mouth
should be at y=300, whereas the middle of the mouth should be at y=360.
In between the positions should be interpolated linearly.
Last, but not least add two eyes to this. They should be at some
reasonable position within the face, but the exact position is up
to you. Both eyes should also change with the smile. The exact
transformation is up to you, but some simple variants are an eye
that is opening or closing (see the hints).
As a starting point, you can use the following code segment,
which is a slightly modified version of the content covered in class.
def movingRectangle():
for frame in range(0,50): #50 frames
canvas = makeEmptyPicture(640,480,white)
addRectFilled(canvas,frame*10,frame*5, 50,50,red)
# Let's have one more
addRectFilled(canvas,100+ int(10 * sin(frame)),4*frame+int(10* cos(frame)),50,50,blue)
writeFrame(frame,"Z:\\",canvas)
def writeFrame(num,directory,framepict):
# expects a directory name terminated with a backslash
# Have to deal with single digit vs. double digit frame numbers differently
framenum=str(num)
if num < 10:
writePictureTo(framepict,directory+"frame0"+framenum+".jpg")
if num >= 10:
writePictureTo(framepict,directory+"frame"+framenum+".jpg")
Creating the final movie file
-
Once you have created all the frames, start Windows Movie Maker in the directory where you created the image files (last item in the "Start" menu).
-
Then go to "Tools->Options->Advanced" and change the "Picture duration" to 0.125 seconds.
-
Choose "Import pictures" from the "Movie Tasks/Capture Video" pane and select all image frames in the file chooser dialog.
-
Then select all pictures in the "Collection" pane, e.g. with Ctrl-A, and drag them to the storyboard pane at the bottom of the window.
-
Then select "Movie Tasks/Finish Movie", give it them the name "Smile" and select the directory where you want to store the movie. This will create the movie in .wmv format.
- You can play this by double-clicking on the file.
Hints
- You can derive the
position of the top left corner of the box around the circle
(which you need to specify to get the circle drawn at the right
place) by subtracting the radius from both coordinates. Reminder:
the radius is half the diameter.
- To generate an eye that opens or closes, you can vary the
"height" of a circle over time. To keep the center of a circle
at the same place, vary the top and bottom y coordinate simultaneously,
e.g. by increasing one by the same amount as you decrease the other.
Please submit that final movie, but not the individual
image files.
What to Turn in
As mentioned previously, you have to add comments with
your own identification (name, student ID, date).
Remember to save the file after you modify it!
How to submit the labtest
As in the labs, you hand in this lab the submit command on the Linux systems that are behind the PRISM lab infrastructure. For details on how to submit, please refer to lab02. However, this time please
submit to lab11, i.e. issue the command
submit 1710 lab11 lab11.py moviefile
Please do not submit the individual images, just the final movie!
Note: You must do all the above steps correctly for receiving
full credit for this labtest.